Based on a talk given at 360iDev 2015. Check out the blog post and video!
Bridging from Objective-C to Swift is great, since that’s the direction all the Apple frameworks need to go.
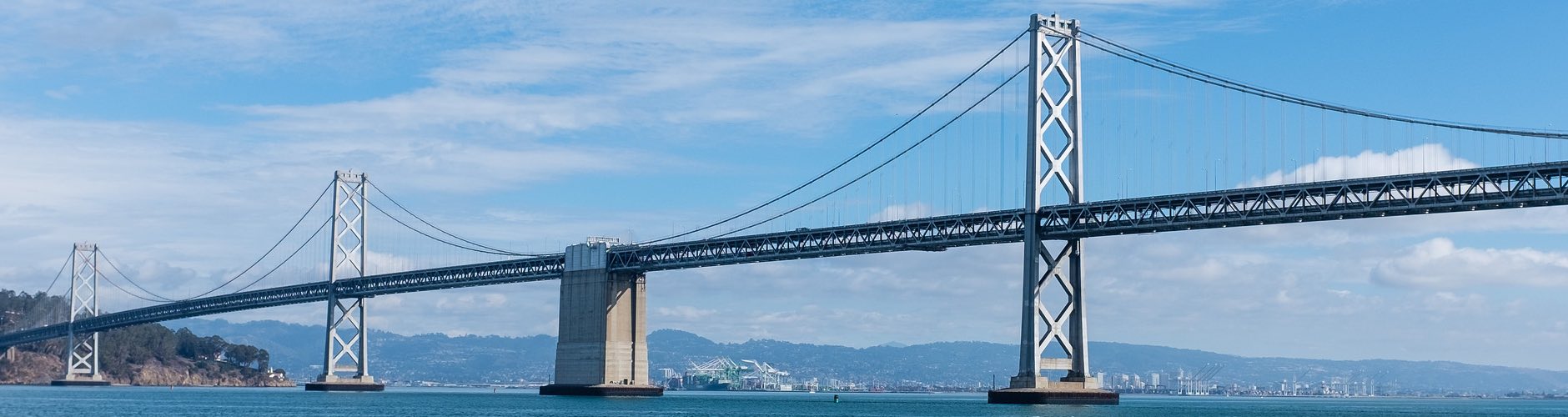
One common gotcha is with support for Swift optionals. Pointers in Objective-C can be nil
but that would mean everything coming from Cocoa would be an optional and need special handling!
To help this along, you add annotations to the types of your properties, arguments, and return values in Objective-C.
Null unspecified: bridges to a Swift implicitly-unwrapped optional. This is the default.
Non-null: the value won’t be
nil
; bridges to a regular reference.Nullable: the value can be
nil
; bridges to an optional.Non-null, but resettable: the value can never be
nil
when read, but you can set it tonil
to reset it. Applies to properties only.
These annotations look slightly different depending on whether you use them as part of the pointer type declaration or the property declaration:
Pointers | Properties |
---|---|
_Null_unspecified | null_unspecified |
_Nonnull | nonnull |
_Nullable | nullable |
N/A | null_resettable |
Some examples:
// property style
@property (nonatomic, strong, null_resettable) NSString *name;
// pointer style
+ (NSArray<NSView *> * _Nullable)interestingObjectsForKey:(NSString * _Nonnull)key;
// these two are equivalent!
@property (nonatomic, strong, nullable) NSString *identifier1;
@property (nonatomic, strong) NSString * _Nullable identifier2;
If you annotate your Objective-C, you’ll get nice typed bridging over in Swift. Even if you never touch Swift, these annotations will show up in the code completion when you write Objective-C. And if you say a method parameter is _Nonnull
and you pass in nil, you’ll get a nice compiler warning.
It’s good practice to start adding these annotations. They’ll help you when using existing API, and then get you up to speed when you start using Swift.